이 게임에는 Bear와 Fish 객체가 등장하며, 이들은 10행 20열의 격자판에서 각각 정해진 규칙에 의해 움직인다.
Bear는 사용자의 키에 의해 왼쪽(a 키), 아래(s 키), 위(d 키), 오른쪽(f 키)으로 한 칸씩 움직이고,
Fish는 다섯 번 중 세 번은 제자리에 있고, 나머지 두 번은 4가지 방향 중 랜덤하게 한 칸씩 움직인다.
게임은 Bear가 Fish를 먹으면(Fish의 위치로 이동) 성공으로 끝난다.
다음은 각 객체의 이동을 정의하는 move( )와
각 객체의 모양을 정의하는 getShape( )을 추상 메소드로 가진 추상 클래스 GameObject이다.
GameObject를 상속받아 Bear와 Fish 클르새를 작성하라.
그리고 전체적인 게임을 진행하는 Game 클래스와 main( ) 함수를 작성하고 프로그램을 완성하라.
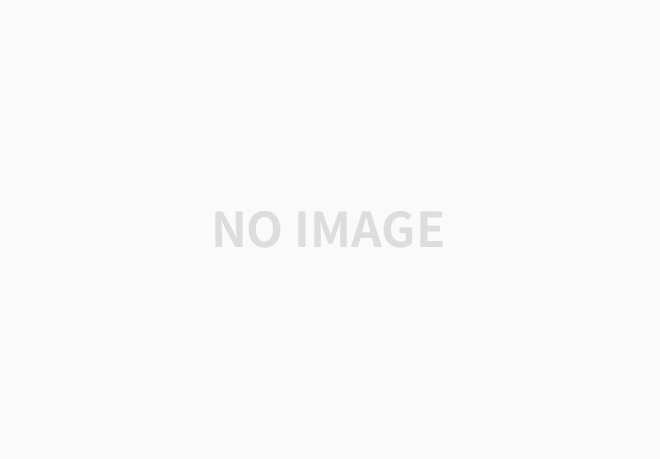
키가 입력될 때마다 Bear와 Fish 객체의 move( )가 순서대로 호출된다.
게임이 진행되는 과정은 다음 그림과 같으며, 게임의 종료 조건에 일치하면 게임을 종료한다.
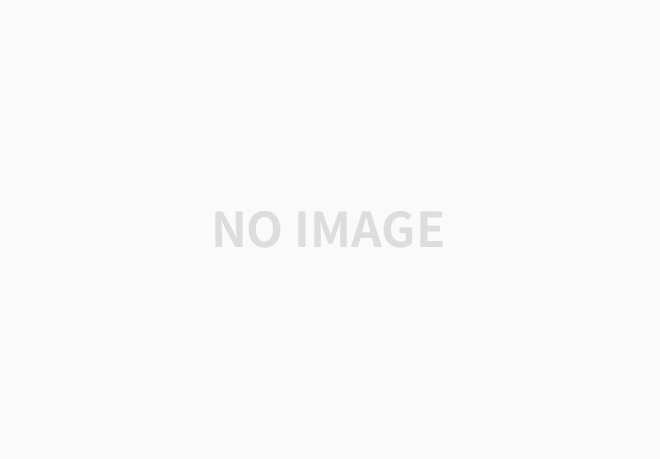
import java.util.Scanner;
public abstract class GameObject { // 추상 클래스
protected int distance; // 한 번 이동 거리
protected int x, y; // 현재 위치(화면 맵 상의 위치)
public GameObject (int startX, int startY, int distance) { // 초기 위치와 이동 거리 설정
this.x = startX;
this.y = startY;
this.distance = distance;
}
public int getX() { return x; }
public int getY() { return y; }
public boolean collide (GameObject p) { // 이 객체가 객체 p와 충돌했으면 true 리턴
if (this.x == p.getX() && this.y == p.getY())
return true;
else
return false;
}
public abstract void move(); // 이동한 후의 새로운 위치로 x, y 변경
public abstract char getShape(); // 객체의 모양을 나타내는 문자 리턴
}
public class Bear extends GameObject {
public Bear (int startX, int startY, int distance) {
super(startX, startY, distance);
}
public void move() {
Scanner scanner = new Scanner(System.in);
System.out.print("왼쪽(a), 아래(s), 위(d), 오른쪽(f) >> ");
char option = scanner.next().charAt(0);
switch(option) {
case 'a':
if (this.y >= distance)
this.y -= this.distance;
break;
case 's':
if (this.x < 10 - this.distance)
this.x += this.distance;
break;
case 'd':
if (this.x >= this.distance)
this.x -= this.distance;
break;
case 'f':
if (this.y < 20 - this.distance)
this.y += this.distance;
break;
}
}
public char getShape() {
return 'B';
}
}
public class Fish extends GameObject {
private int fiveMove [];
private int fiveCheck;
public Fish (int startX, int startY, int distance) {
super(startX, startY, distance);
fiveMove = new int [5];
fiveCheck = 0;
}
public void fiveMoving() {
int check;
while (true) {
check = 0;
for (int i = 0; i < fiveMove.length; i++) {
fiveMove[i] = (int)(Math.random() * 5); // 0:왼쪽, 1:아래, 2:위, 3:오른쪽, 4:제자리
if (fiveMove[i] == 4)
check++;
}
if (check == 3)
break;
}
}
public void move() {
if (fiveCheck == 5) {
fiveCheck = 0;
fiveMoving();
}
else if (fiveCheck == 0)
fiveMoving();
switch (fiveMove[fiveCheck]) {
case 0:
if (this.y >= this.distance)
this.y -= this.distance;
break;
case 1:
if (this.x >= this.distance)
this.x -= this.distance;
break;
case 2:
if (this.x < 10 - this.distance)
this.x += this.distance;
case 3:
if (this.y < 10 - this.distance)
this.y += this.distance;
case 4:
break;
}
fiveCheck++;
}
public char getShape() {
return '@';
}
}
public class Game {
private Bear bear;
private Fish fish;
Game() {
bear = new Bear(0, 0, 2);
fish = new Fish(6, 6, 1);
}
public void run() {
while(true) {
System.out.println();
for(int i = 0; i < 10; i++) {
for(int j = 0; j < 20; j++) {
if (bear.getX() == i && bear.getY() == j)
System.out.print(bear.getShape());
else if (fish.getX() == i && fish.getY() == j)
System.out.print(fish.getShape());
else
System.out.print("-");
}
System.out.println("");
}
bear.move();
fish.move();
if (bear.collide(fish)) {
for (int i = 0; i < 10; i++) {
for (int j = 0; j < 20; j++) {
if (bear.getX() == i && bear.getY() == j)
System.out.print(bear.getShape());
else
System.out.print("-");
}
System.out.println();
}
finish();
}
}
}
public void finish() {
System.out.println("Bear Wins!!");
System.exit(0);
}
public static void main(String[] args) {
Game game = new Game();
System.out.println("**Bear의 Fish 먹기 게임을 시작합니다.**");
game.run();
}
}